Pi-Rat
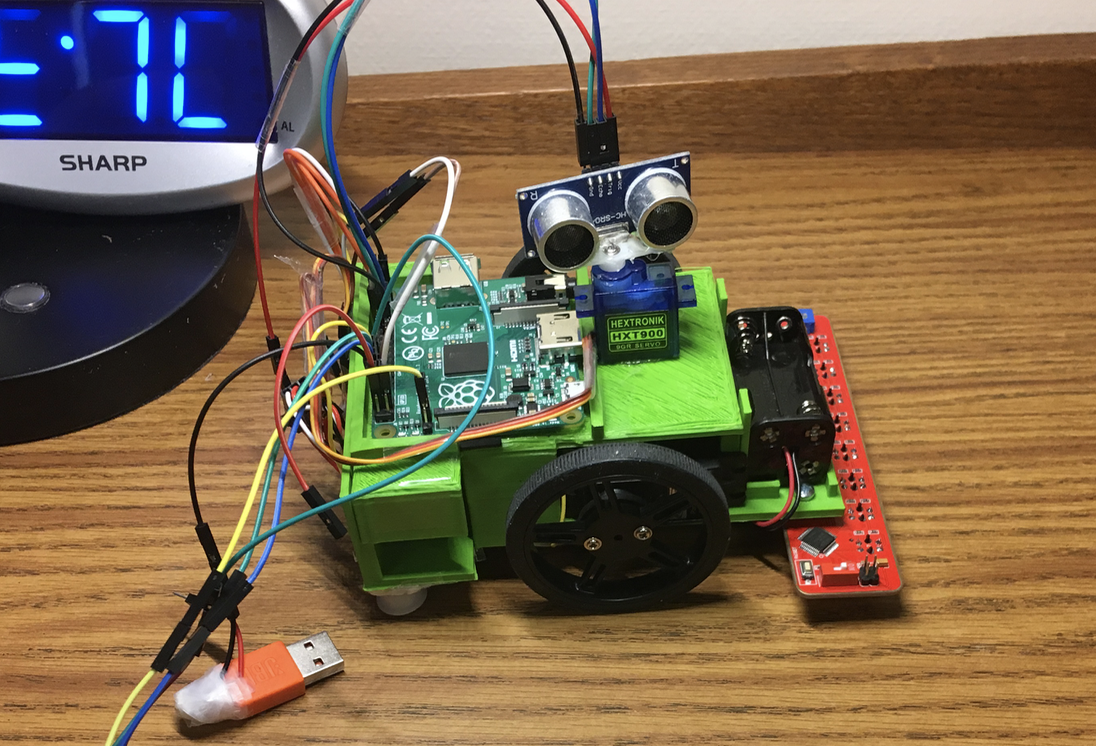
Click the image or here to see the project's repository (checkout readme.md and proposal.md first)!
What is Pi Rat?
Pi Rat is an autonomous maze solving robot that is powered by a bare-metal programmed Raspberry Pi. By bare-metal, I mean that the Raspberry Pi has no operating system, and code is running directly on the ARM processor (Raspberry Pi A+). In order to run code on the bare-metal Raspberry Pi, I need to first write it on my computer, compile it, and then load it onto the Raspberry Pi (which is running a bootloader that waits for input) over the UART through the bootloader.
What Technology does Pi Rat Utilize?
- Raspberry Pi, bare-metal, programmed completely in C.
- Pointers
- Bitwise Manipulation
- Logic Analyzer
- gcc/gdb/valgrind
- Makefiles
- GPIO and Timer Interrupts
- PWM I/O
- UART communication
- GPIO
- Bootloader
- Bit Banging
- I2C
- Fusion360
- 3D Printing
- Laser Cutting
- Line Following Module
- Servo Motor
- Continuous Rotation Servo Motor (CRSM)
- Hall Effect Sensor (to detect CRSM position)
- Ultrasonic Distance Sensor
- Depth First Search
- SLAM
- Negative Feedback Control Loop
- malloc
- printf
- c structs
What Are the Coolest Parts of Pi Rat?
The single thing I am most proud of in this project is how I was able to build it entirely from the ground up. libmypi.a
is a compiled library
that I wrote myself. It includes c modules like printf
, malloc
, string
and gpio
which I implemented myself
over the course of 8 weeks in cs107e. Furthermore, I also implemented the modules which interface Pi Rat specific low level features, like servo motor control or reading inputs
from the ultrasonic distance sensor. And finally, I even designed the robot's physical design (I CADded it's chassis from scratch in Fusion360) as well as the modular
maze (using laser cut duron and scrap cardboard I found). I truly feel that I can say I own this project, and understood every aspect of it from a rigorous, ground up
perspective. If you were to recursively ask, "how does this work," I could bring you to the base case.
What Were the Biggest Challenges of Pi Rat?
The biggest challenge was the number of moving parts within the project combined with the fact that I had to build each part up from the ground up. In order to efficiently work with so many moving parts, I had to build up proper organization and structure into the project, before I could begin implementing things. However, in order to know what the best structure was, I often felt that I needed to implement things first to learn more about them. That's an issue, as you can see. To help me organize these thoughts a little better, I turned to an object oriented approach. A servo thus became an object, which I would be able to independently abstract away and implement, and then use.
However, abstracting different things away wasn't simple, because many of these things were deeply intertwined. For example, I had to both read PWM inputs from the Hall Effect Sensor to determine wheel positions and write them to control the servos. But the issue was I was only working in a single threaded process, and my implementation of PWM writing ended up interfering with my implementation of PWM reading, and so I needed to properly time each action so as they would not conflict, implementing my own, basic process scheduler.
More challenges lay within that many moving parts were not even based in code. For example, when trying to communicate with the line follower module, it would often seem to work inconsistently for no apparent reason. As it turns out, it's because I connected a ground pin to the wrong line. Little bugs like this happened often, and hardware bugs added another layer of difficulty in getting things working.
I talk about more challenges and go into technical detail in the repository
What did I learn from Pi Rat?
- Organization is huge. It's critical and worthwhile to think deeply about the structure of the entire project because by you can solve a challenge by phrasing it in a different context that makes it much more doable.
- Hacking is sometimes cool, but often not good. In fact, you should only hack when you absolutely have to. Because while it may save time in the short run, it will almost always come to bite you in the end. Do things right the first time, and fix things from the source.
- Transparent code execution is so critical to efficient debugging. You really do need to see the entire picture when fixing your code, and it really is worth the extra difficulty and time to use a debugger. Because otherwise, while you may be able to determine the mechanism behind the actions you see, more often than not, you will have an incorrect understanding of what is going on and you will waste unnecessary time and effort on debugging excursions based on incorrect beliefs.
- Checkout the readme & proposal in the repository for more